注記
完全なサンプルコードをダウンロードするには、こちらへ。または、Binder経由でブラウザでこの例を実行することもできます。
SIFT特徴検出器と記述子抽出器#
この例では、SIFT特徴検出とその記述アルゴリズムを示します。
尺度不変特徴変換(SIFT)[1]は1999年に発表され、 「画像のスケーリング、平行移動、回転に対して不変であり、照明変化やアフィン変換、3D投影に対しても部分的に不変である」[2]という約束から、現在でも最も人気のある特徴検出器の1つです。最大の欠点は実行時間で、ORBよりも「2桁」[3]遅いと言われており、リアルタイムアプリケーションには適していません。
参考文献#
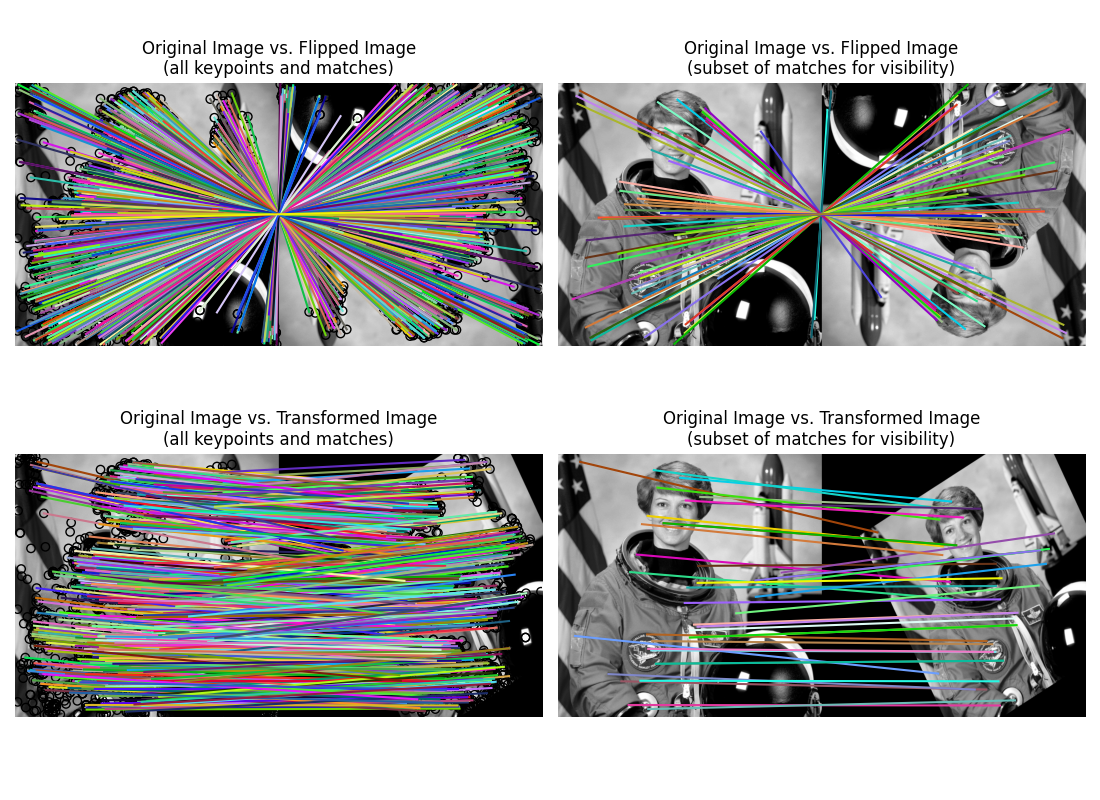
import matplotlib.pyplot as plt
from skimage import data
from skimage import transform
from skimage.color import rgb2gray
from skimage.feature import match_descriptors, plot_matched_features, SIFT
img1 = rgb2gray(data.astronaut())
img2 = transform.rotate(img1, 180)
tform = transform.AffineTransform(scale=(1.3, 1.1), rotation=0.5, translation=(0, -200))
img3 = transform.warp(img1, tform)
descriptor_extractor = SIFT()
descriptor_extractor.detect_and_extract(img1)
keypoints1 = descriptor_extractor.keypoints
descriptors1 = descriptor_extractor.descriptors
descriptor_extractor.detect_and_extract(img2)
keypoints2 = descriptor_extractor.keypoints
descriptors2 = descriptor_extractor.descriptors
descriptor_extractor.detect_and_extract(img3)
keypoints3 = descriptor_extractor.keypoints
descriptors3 = descriptor_extractor.descriptors
matches12 = match_descriptors(
descriptors1, descriptors2, max_ratio=0.6, cross_check=True
)
matches13 = match_descriptors(
descriptors1, descriptors3, max_ratio=0.6, cross_check=True
)
fig, ax = plt.subplots(nrows=2, ncols=2, figsize=(11, 8))
plt.gray()
plot_matched_features(
img1,
img2,
keypoints0=keypoints1,
keypoints1=keypoints2,
matches=matches12,
ax=ax[0, 0],
)
ax[0, 0].axis('off')
ax[0, 0].set_title("Original Image vs. Flipped Image\n" "(all keypoints and matches)")
plot_matched_features(
img1,
img3,
keypoints0=keypoints1,
keypoints1=keypoints3,
matches=matches13,
ax=ax[1, 0],
)
ax[1, 0].axis('off')
ax[1, 0].set_title(
"Original Image vs. Transformed Image\n" "(all keypoints and matches)"
)
plot_matched_features(
img1,
img2,
keypoints0=keypoints1,
keypoints1=keypoints2,
matches=matches12[::15],
ax=ax[0, 1],
only_matches=True,
)
ax[0, 1].axis('off')
ax[0, 1].set_title(
"Original Image vs. Flipped Image\n" "(subset of matches for visibility)"
)
plot_matched_features(
img1,
img3,
keypoints0=keypoints1,
keypoints1=keypoints3,
matches=matches13[::15],
ax=ax[1, 1],
only_matches=True,
)
ax[1, 1].axis('off')
ax[1, 1].set_title(
"Original Image vs. Transformed Image\n" "(subset of matches for visibility)"
)
plt.tight_layout()
plt.show()
スクリプトの総実行時間:(0分6.490秒)